Looking to add a Chat SDK to your Android mobile app? Start with Crisp today and elevate your customer support with our Android Chat widget — Install it in just a few steps.
Looking for the extensive Java documentation? It is available here.
Video Tutorial
Installation
1. Get your Website ID
Go to your Crisp Dashboard, and copy your Website ID:
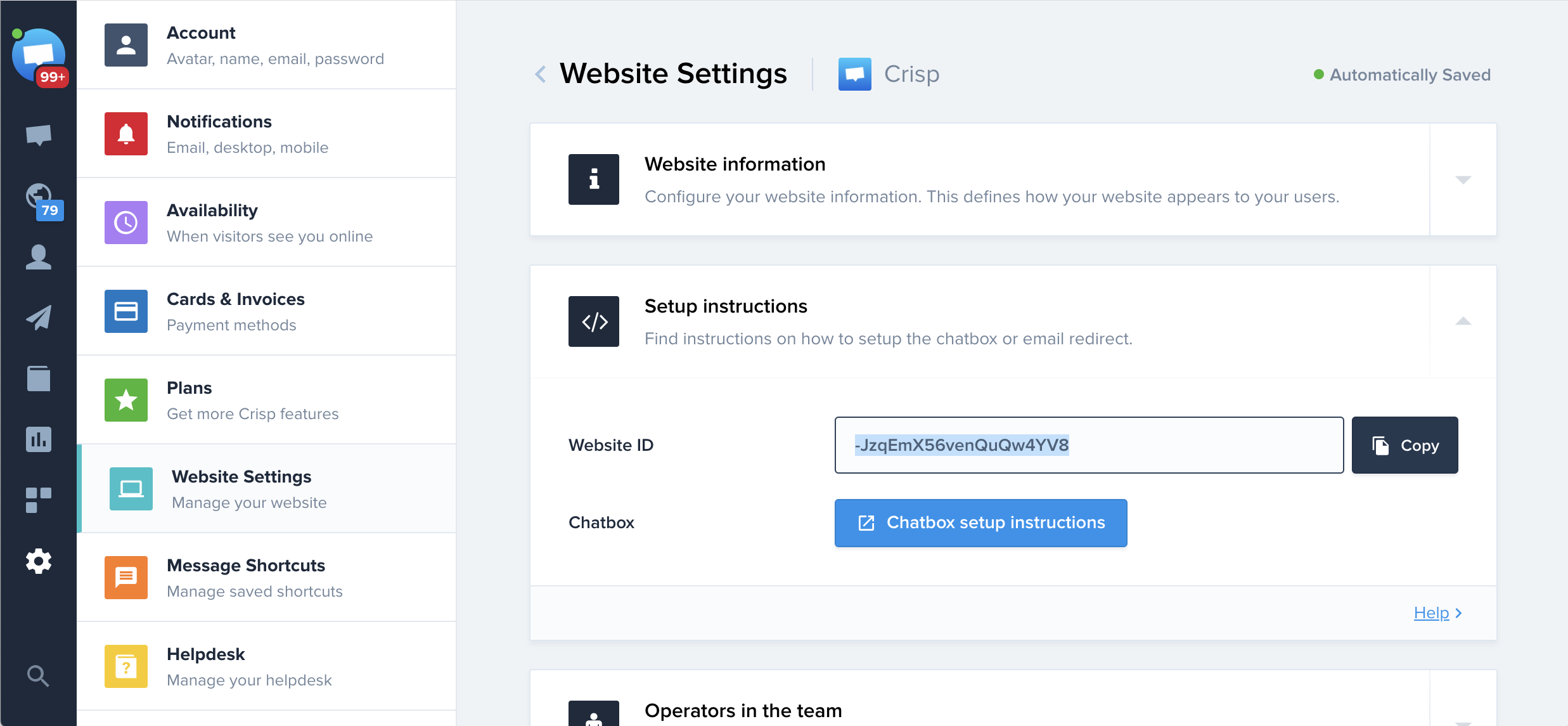
2. Add Crisp dependency
Add the Crisp Android Chat SDK in your dependencies:
dependencies {
implementation 'im.crisp:crisp-sdk:2.0.0beta1'
}
3. Setup multidex
Configure your app for multidex:
android {
defaultConfig {
multiDexEnabled true
}
}
dependencies {
// If you're using AndroidX
implementation 'androidx.multidex:multidex:2.0.1'
// If you're not using AndroidX
implementation 'com.android.support:multidex:1.0.3'
}
4. Initiate the application class
Initialize the library in your Application subclass:
public class Initializer extends MultiDexApplication {
@Override
public void onCreate() {
super.onCreate();
// Replace it with your WEBSITE_ID
// Retrieve it using https://app.crisp.chat/website/[YOUR_WEBSITE_ID]/
Crisp.configure(getApplicationContext(), "7598bf86-9ebb-46bc-8c61-be8929bbf93d");
}
}
5. Include Crisp in your activity
You can for instance start Crisp after a click on a button:
Intent crispIntent = new Intent(this, ChatActivity.class);
startActivity(crispIntent);
Availables APIs
Java APIs
The extensive Java documentation is available here.
Crisp.configure(Context applicationContext, String websiteID, @Nullable String tokenID)
— Configures Crisp SDK with your websiteID and optionally your user's tokenIDCrisp.setTokenID(Context applicationContext, @Nullable String tokenID)
— Assigns the next session with a tokenIDCrisp.resetChatSession(Context applicationContext)
— Reset the sessionCrisp.getSessionIdentifier(Context)
— Returns the sessionIDCrisp.setUserEmail(String email)
— Sets the user email (note: if email is invalid, this method will return false and the value will not be set)Crisp.setUserPhone(String phone)
— Sets the user phone (note: if phone is invalid, this method will return false and the value will not be set)Crisp.setUserNickname(String nickname)
— Sets the user nameCrisp.setUserAvatar(String avatar)
— Sets the user avatar (note: if avatar is invalid, this method will return false and the value will not be set)Crisp.setUserCompany(Company company)
— Sets the user companyCrisp.setSessionBool(String key, boolean value)
— Sets a session data booleanCrisp.setSessionInt(String key, int value)
— Sets a session data integerCrisp.setSessionString(String key, String value)
— Sets a session data stringCrisp.setSessionSegment(String segment, boolean overwrite)
— Sets a session segment and optionally overwrite existing ones (default is false)Crisp.setSessionSegments(List<String> segments, boolean overwrite)
— Sets a collection of session segments and optionally overwrite existing ones (default is false)Crisp.pushSessionEvent(SessionEvent event)
— Pushes a eventCrisp.pushSessionEvents(List<SessionEvent> events)
— Pushes a collection of eventsCrisp.searchHelpdesk(Context applicationContext)
— Opens the Helpdesk search tabCrisp.openHelpdeskArticle(Context applicationContext, String id, String locale, @Nullable String title, @Nullable String category)
— Displays the targeted article (optionally sets a title and category)
Important notes
Calls to
setUser
, setSession
and pushSessionEvent
methods are performed right away if the chat is already ongoing, when the session is joined, or if you call either of configure
(with a different websiteID than the current one) or resetChatSession
.Calls to
helpdesk
methods are performed right away if the chat is already ongoing, on the next opening, or if you call either of configure
(with a different websiteID than the current one), resetChatSession
or any other helpdesk
methods.Calling
configure(Context, String)
or configure(Context, String, String)
with a null
tokenID value will set it to null
. This means that calls to setTokenID
must be done after configure
and before starting ChatActivity
, otherwise the session will be created without it and you will need to call resetChatSession
afterwards. Calls to configure
and setTokenID
are ignored if the chat is already ongoing.